728x90
반응형
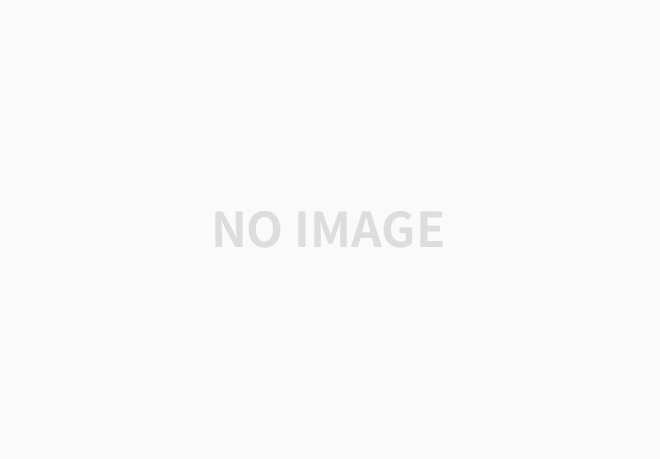
- 주제
>> 인스턴스 생성자(클래스의 인스턴스 사용, 추상클래스와 하위클래스)
- 공부내용
인스턴스 생성자란?
: new 식으로 형식의 새 인스턴스를 만들 때, 실행되는 코드를 지정하기 위해 선언하는 것이다.
<예제 1>
좌표를 나타내는 Coords 클래스를 정의하고, 그 클래스의 인스턴스를 사용한다.
class Coords
{
public int X { get; set; }
public int Y { get; set; }
public Coords() //매개변수 없는 생성자
: this(0, 0) //this 키워드를 사용하여 Coords(int x, int y)생성자를 호출 -> (0,0)으로 초기화
{ }
public Coords(int x, int y) //X와 Y 속성을 초기화하는 생성자
{
X = x;
Y = y;
}
public override string ToString() => $"({X},{Y})"; //좌표를 문자열로 변환하여 반환하는 메서드
}
class Example
{
static void Main()
{
var p1 = new Coords();
Console.WriteLine($"Coords #1 at {p1}"); // Output: Coords #1 at (0,0)
var p2 = new Coords(5, 3);
Console.WriteLine($"Coords #2 at {p2}"); // Output: Coords #2 at (5,3)
//ToString()메서드가 자동으로 호출되어 좌표가 문자열로 변환되어 출력됨
}
}
<예제 2>
Shape 추상클래스와 그것을 구현하는 하위클래스들을 정의하고, 이를 사용하여 원과 원기둥의 면적을 계산한다.
abstract class Shape
{
public const double pi = Math.PI;
protected double x, y; //보호된 멤버 변수를 정의
public Shape(double x, double y) //생성자는 두개의 매개변수를 받아 x, y를 초기화
{
this.x = x;
this.y = y;
}
public abstract double Area(); //면적을 계산하는 메서드(하위클래스에서 구현 필요)
}
class Circle : Shape //Shape 클래스의 하위클래스
{
public Circle(double radius) //원의 반지름을 받는 생성자 정의
: base(radius, 0) //원은 높이가 없으므로 y를 0으로 설정
{ }
public override double Area() => pi * x * x; //Area메서드를 구현하여 원의 면적을 계산
}
class Cylinder : Circle //Circle 클래스의 하위클래스
{
public Cylinder(double radius, double height) //원기둥의 반지름과 높이를 받는 생성자 정의
: base(radius)
{
y = height; //원기둥의 높이 y를 초기화
}
public override double Area() => (2 * base.Area()) + (2 * pi * x * y); //원기둥 전체 면적 계산
}
class Example
{
static void Main()
{
double radius = 2.5; //반지름 값 설정
double height = 3.0; //높이 값 설정
var ring = new Circle(radius); //원의 면적 계산하여 출력
Console.WriteLine($"Area of the circle = {ring.Area():F2}");
// Output: Area of the circle = 19.63
var tube = new Cylinder(radius, height); //원기둥의 면적 계산하여 출력
Console.WriteLine($"Area of the cylinder = {tube.Area():F2}");
// Output: Area of the cylinder = 86.39
}
}
728x90
반응형
'🌐 유니티 (Unity)' 카테고리의 다른 글
Day 26 - Player Input System을 이용한 이동 및 점프 구현 (10) | 2024.05.14 |
---|---|
Day 25 - 아크탄젠트(마우스 위치로 회전, 총알 발사 방향 설정 등) (0) | 2024.05.13 |
Day 23 - 탑뷰게임 만들기(InputField) (0) | 2024.05.10 |
Day 22 - 탑뷰게임 만들기(플레이어를 따라가는 카메라) (0) | 2024.05.09 |
Day 21 - C# (Nullable, StringBuilder) (0) | 2024.05.08 |